We now arrived at the last post of this series about Domain-Driven Design (DDD) patterns…
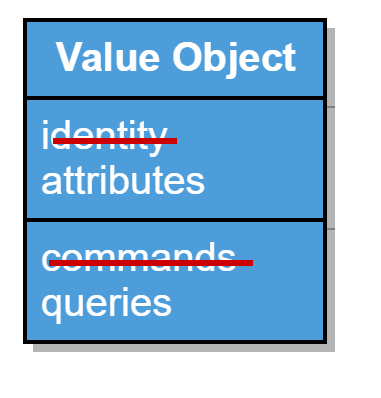
DDD Concepts and Patterns – Value Object and Factory
This post is about the value object pattern and the factory pattern which are tactical patterns in domain driven design (DDD). Value objects define the second kind of domain objects besides entities. Their main characteristic is immutability: Attributes of a value object never change.
Factories are responsible for creating new objects and validate them. They help us identify and separate this functionality from the other parts of a domain object.
Value Objects
Value objects do have attributes and methods as entities. Attributes of value objects are immutable though which implies that methods of value objects can only be queries, never commands that change the internal state of an object. We can pass value objects to clients through getters for example without worrying that they change them.
Many objects can be modeled as value objects instead of entities because they are defined through their attributes. These objects measure, quantify or describe things in the domain model. Because they are so easy to handle we should model as many value objects as possible.
No identity, no lifecycle
Value objects don’t have an identity that is used to compare them. We compare them by their attributes. When all attributes of a value object match another one they are considered equal. Therefore we can construct a value object from its attributes when needed and destroy it afterward.
Without the burden of maintaining an identity (create a unique id, associate it with other entities) value objects are a lot easier to handle than entities.
Example
An example of a value object is the material of a tabletop. The tabletop’s main material can be wood, stone or steel for example. Instead of adding attributes for kind (wood, steel), name (oak, chromium steel) and thickness directly to the table object let us create a value object called material.
Now the material is explicitly defined and named. We can interact with the table without thinking about the details of the material.
Maybe you decide the replace the tabletop with another after some years. However, because value objects are immutable, the material object cannot be changed. We replace it with the new one. Just like in the physical world, stone can’t be transformed into wood magically.
In code, the replacement boils down to setting new attributes and save them to persistence. If we modeled the material as an entity with identity, we first would need to delete the old material, generate a new id and associate it with the table object.
Now on to the second pattern of this post, the factory.
Factory
The factory pattern in DDD can be seen as a super pattern for the Gang of Four (GoF) creational patterns. Factories are concerned with creating new entities and value objects. They also validate the invariants for the newly created objects. We can place a factory on the entity or value object itself or an independent object.
Factories that are placed on the same object they create are either factory methods or prototype methods. The factory method creates a completely new object from the method parameters. The prototype method uses an existing instance to derive a new object.
When the creation logic is complex or has dependencies that are not needed by the created object it is best to create a separate factory. This factory could then provide multiple ways to create new instances.
Wrap Up / Final Thoughts
Value objects simplify our lives by releasing us from thinking about mutated state just like in the functional programming style. By combining them with entities, we can get a simple but powerful model within our code.
Factories draw a clear line between creation logic and other concerns in the code. When we name them explicitly and consistently, readability of our code should be improved.
[Eva04] Eric Evans: Domain-Driven Design – Tackling Complexity in the Heart of Software (homepage)